Just the other day I sat at a customer, and they wanted a web application to present data, from there Mule integration application. The data should be presented to some users that should be able to update a few fields in the dataset. The Customer wanted a simple solution, and not any new servers such as tomcat or Apache to run the web application because they don’t have personal to run and maintain these servers.
So I thought it must be possible to use Mule ESB, to deliver some simple HTML, JavaScript, CSS pages and then use AJAX to acquire the necessary data through a call to an HTTP connector in a Mule application. So here is what I did. First I created the flow to supply the static pages HTML, JavaScript, CSS and images here I used a simple “HTTP Connector” as an inbound endpoint and an “HTTP Static Resource Handler” as a processor.
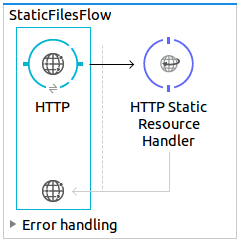
Here is the XML for the flow.
<?xml version="1.0" encoding="UTF-8"?>
<mule xmlns:context="http://www.springframework.org/schema/context"
xmlns:http="http://www.mulesoft.org/schema/mule/http"
xmlns="http://www.mulesoft.org/schema/mule/core"
xmlns:doc="http://www.mulesoft.org/schema/mule/documentation"
xmlns:spring="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-current.xsd http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd http://www.mulesoft.org/schema/mule/http http://www.mulesoft.org/schema/mule/http/current/mule-http.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-current.xsd">
<http:listener-config name="HTTP_Listener_Configuration" host="0.0.0.0" port="8081" doc:name="HTTP Listener Configuration"/>
<context:property-placeholder location="dev.properties"/>
<flow name="muleaswebserverFlow">
<http:listener config-ref="HTTP_Listener_Configuration" path="/*" allowedMethods="GET" doc:name="HTTP"/>
<http:static-resource-handler resourceBase="${app.path}/web" defaultFile="index.html" doc:name="HTTP Static Resource Handler"/>
</flow>
</mule>
As you can see in the flow diagram above, first we make a new Connector
Configurations, where we just use the default values for port: 8081
and Host: All Interfaces [0.0.0.0] (Default) then we specify the path:
/*
and Allowed Methods: GET We now configure the “HTTP Static
Resource Handler” by setting the Resource Base and the default file
name; I made a folder called the web under the main/resources
folder. And in the HTTP Static Resource Handler under Resource Base:
set the path to the new web folder, and as default file name I called
my file index.html, but you can call it anything. (see screen dump
below for my structure)
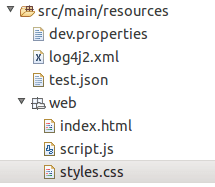
If you have trouble getting other files than the index.html (or default file name) you have properly forgotten to set the * in the path in the HTTP connector. Now your are set to go displaying static content from your Mule ESB, but we can make it a little more exciting, in the same Mule application as the one who delivers the static content we can make one or more flows there can be called with some AJAX. There are many sites online there explain how to use AJAX from at JavaScript Framework as JQuery or where you implement it in plain JavaScript code, just google it. Here is an example link for a JQuery example, or if you want to write it yourself an example in plain JavaScript Here is my AJAX Flow implemented with an ordinary HTTP Connector.
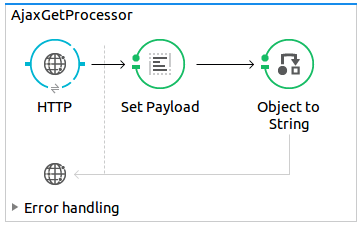
<?xml version="1.0" encoding="UTF-8"?>
<mule xmlns:file="http://www.mulesoft.org/schema/mule/file"
xmlns:db="http://www.mulesoft.org/schema/mule/db"
xmlns:json="http://www.mulesoft.org/schema/mule/json"
xmlns:tracking="http://www.mulesoft.org/schema/mule/ee/tracking"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:http="http://www.mulesoft.org/schema/mule/http"
xmlns="http://www.mulesoft.org/schema/mule/core"
xmlns:doc="http://www.mulesoft.org/schema/mule/documentation"
xmlns:spring="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-current.xsd http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd http://www.mulesoft.org/schema/mule/http http://www.mulesoft.org/schema/mule/http/current/mule-http.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-current.xsd http://www.mulesoft.org/schema/mule/db http://www.mulesoft.org/schema/mule/db/current/mule-db.xsd http://www.mulesoft.org/schema/mule/ee/tracking http://www.mulesoft.org/schema/mule/ee/tracking/current/mule-tracking-ee.xsd http://www.mulesoft.org/schema/mule/file http://www.mulesoft.org/schema/mule/file/current/mule-file.xsd http://www.mulesoft.org/schema/mule/json http://www.mulesoft.org/schema/mule/json/current/mule-json.xsd">
<http:listener-config name="HTTP_Listener_Configuration" host="0.0.0.0" port="8081" doc:name="HTTP Listener Configuration"/>
<context:property-placeholder location="dev.properties"/>
<file:connector name="File" outputPattern="test.json" readFromDirectory="/home/jack/tools/anypoint/workspace/muleaswebserver/muleaswebserver/src/main/resources/web" autoDelete="false" streaming="true" validateConnections="true" doc:name="File"/>
<file:file-to-string-transformer name="File_to_String" doc:name="File to String"/>
<flow name="StaticFilesFlow">
<http:listener config-ref="HTTP_Listener_Configuration" path="/*" allowedMethods="GET" doc:name="HTTP"/>
<http:static-resource-handler resourceBase="${app.path}/web" defaultFile="index.html" doc:name="HTTP Static Resource Handler"/>
</flow>
<flow name="AjaxGetProcessor">
<http:listener config-ref="HTTP_Listener_Configuration" path="/ajaxprocessor/" allowedMethods="GET" doc:name="HTTP"/>
<set-payload value="#[Thread.currentThread().getContextClassLoader().getResourceAsStream('test.json')]" encoding="UTF-8" mimeType="application/json" doc:name="Set Payload"/>
<object-to-string-transformer doc:name="Object to String"/>
</flow>
</mule>
You just make a normal HTTP connector as inbound endpoint then I use a set payload to read a JSON file from the resource folder. The file is converted from bufferedinputStream to a string by using the “Object to String” converter then you send it back as a response to the AJAX call.
That’s pretty much what there is to it if you want to download the
whole Anypoint project then download it from
GitHub. The project
contains mule flows, HTML, CSS, JavaScript files - all there should be
for you to start it and in a browser go to localhost:8081
.